Install OpenCV on Raspberry Pi: The only guide you need
OpenCV is often used to promote Raspberry Pi capacities. Even if the Raspberry Pi is a cheap piece of hardware, showing it with a camera, using artificial intelligence and electronics is pretty impressive. But before doing all of this, you have to set it up correctly, and it isn’t straightforward for beginners. That’s why I wrote this tutorial, to help you to get started with OpenCV.
The easiest way to install OpenCV on a Raspberry Pi is to use the package manager (APT). But to get a recent version, using PIP is recommended, as the version available with apt is generally outdated.
Let’s start with a brief introduction to OpenCV and its main features, and then I’ll show you how to install it on your Raspberry Pi and create your first scripts with it.
By the way, if you get overwhelmed as soon as Python is required for a project, I recommend checking out my e-book “Master Python on Raspberry Pi“. It will guide you step-by-step to learn the essential concepts (and only the essential concepts) required to achieve any project in the future. Raspberry Pi without Python is like a car without an engine, you miss all the fun parts. Get 10% off by downloading it today!
What is OpenCV?
OpenCV is an open-source library, compatible with languages like Python or C++, which allows you to build computer vision and machine learning projects.
By the way, CV stands for “Computer Vision”. In short, it’ll provide you with interesting functions to manipulate images and videos. If you are reading this, you have probably already seen some cool projects using it.
Typical examples of OpenCV use are image manipulation (I’ll give you some basic code to do this later), object detection (recognizing a specific type of object, like a face or pet), or object tracking (a robot can follow you, for example).
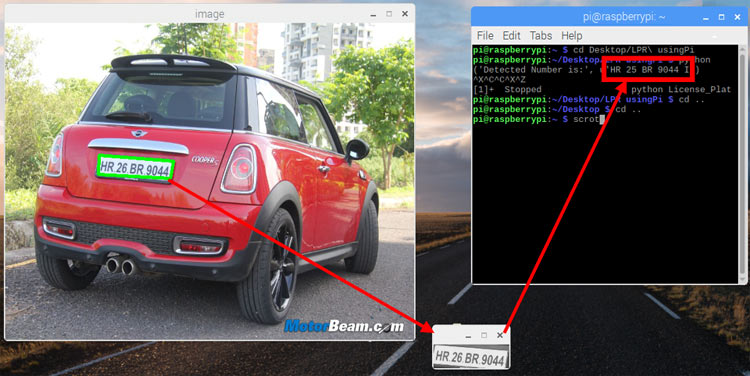
A classic OpenCV project will use other features from the Raspberry Pi, like the camera module and the GPIO pins. You can for example have the camera detect when your car shows up in front of the garage (object detection), find the license plate, and read the number on it (text recognition). If it matches, you can send a signal to the GPIO pins (or a smart device) to open the garage door.
Well, this is not exactly the easiest project to get started with OpenCV, but it gives you an idea. First, let’s learn how to install the software on your system.
Install OpenCV on Raspberry Pi
Get Raspberry Pi OS
The easiest way to use OpenCV on Raspberry Pi is to use the official operating system: Raspberry Pi OS. It comes with Python preinstalled, so you’ll just need to add the OpenCV library before creating your first script.
If you need some help installing Raspberry Pi OS, you can click on the link to read my tutorial where I explain everything step-by-step. A desktop version is probably better, as we’ll test it with images and the camera module, but pick whatever version makes sense for you.
Whether you are installing it from scratch, make sure you do the following steps before going further:
- Connect to the Internet (via Ethernet or Wi-Fi).
- Do the system updates:
sudo apt update
sudo apt upgrade - Enabling SSH and/or VNC is probably a good idea if you want to follow this tutorial from your computer directly.
Once ready, you can move to the OpenCV installation.
If like me, you always mix the languages syntax, download my cheat sheet for Python here!
Download now
Method 1 – Install OpenCV via APT
Installing OpenCV on Raspberry Pi OS can be done with apt directly. Open a terminal and type this command:sudo apt install python3-opencv
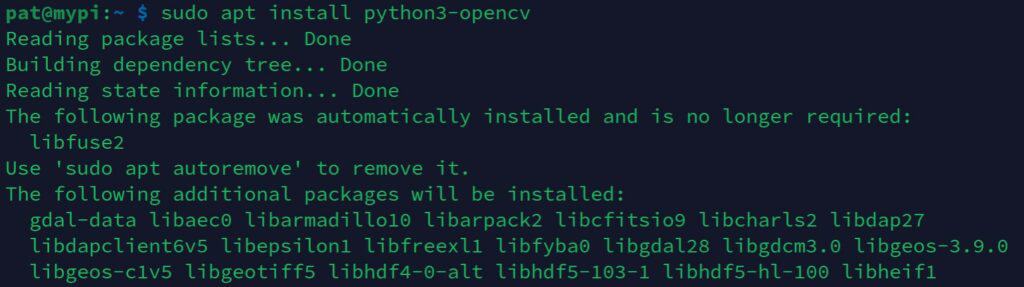
I’ve seen many tutorials where they do the installation from the source code, but it’s pretty complicated, and you don’t need to install it that way anymore. This command will install all the dependencies, and it will work directly after that.
The issue with this method, however, is that you’ll most likely get an outdated version of OpenCV. Check the next method to get something more recent.
I’m going to use Python for this tutorial, as it’s the easiest way to start (everything is ready for Python on Raspberry Pi OS), but OpenCV can also be used with other languages. For example, install libopencv-dev instead to use it with C++.
Are you a bit lost in the Linux command line? Check this article first for the most important commands to remember and a free downloadable cheat sheet so you can have the commands at your fingertips.
Method 2 – Use PIP to install OpenCV
At the time of writing, the version of OpenCV available via APT is 4.5.1 (released in 2020), while the latest version available on the official website is 4.8 (summer 2023). Which means you won’t get the latest bug fixes and improvements if you use APT.
Join Our Community!
Connect, learn, and grow with other Raspberry Pi enthusiasts. Support RaspberryTips and enjoy an ad-free reading experience. Get exclusive monthly video tutorials and many other benefits.
Learn moreYou may also have incompatibilities with other libraries you’ll be using with OpenCV.
That’s why using PIP, especially when working in Python, is recommended.
Warning, though, don’t mix the two options.
So if you already installed OpenCV by following the previous method, remove it with APT before anything else:sudo apt remove python3-opencv
You can then use PIP to get the latest version:pip install opencv-python
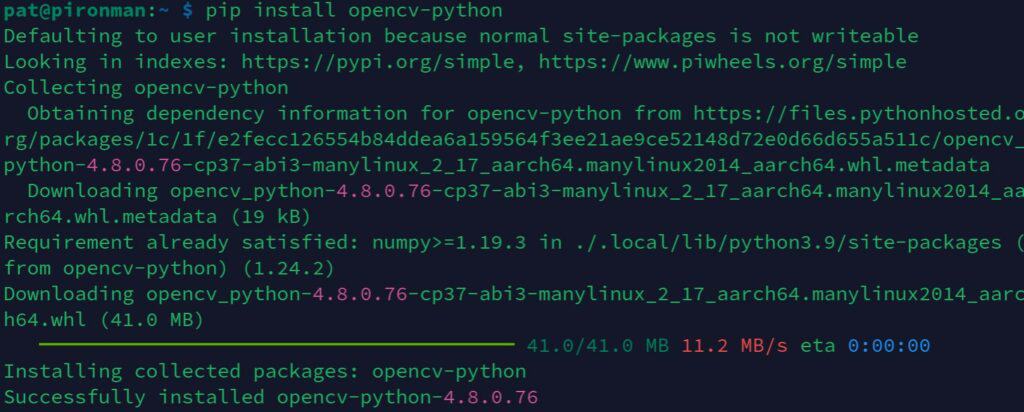
It might take a while depending on your hardware and system version, just be patient. It took a few seconds on my Pironman setup (RPI OS 64), but a 10+ minutes on my fresh installation with Raspberry Pi OS 32-bits.
If you get an error, you might need to run this command to upgrade pip and wheel:pip install --upgrade pip setuptools wheel
Then run the installation again:pip install opencv-python
And if you still get an error after that, it’s probably because you try to install a version, that is too recent and not yet compatible with your system.
In this case, you can try to specify an older version with something like:pip install opencv-python==4.7.0.72
The list of the latest versions available with pip is available here.
Test OpenCV with Python
Once OpenCV is installed on your Raspberry Pi, create a quick test to make sure it’s working as expected. Create a new file and copy the following lines into it:import cv2
print(cv2.version)
When you run this script, it should display the current OpenCV version installed on your system:
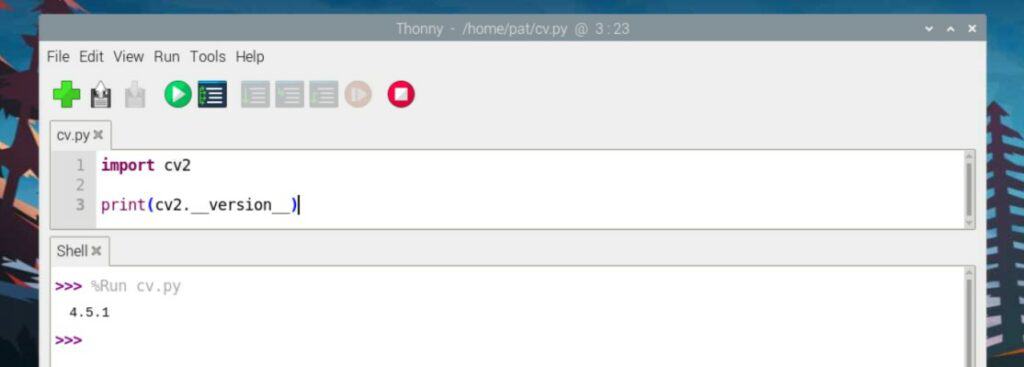
You’ll get different results depending on the installation method followed, but the code should be the same.
If you experience any error at this point, make sure to double-check the previous steps, as you probably missed something.
I’m using Thonny as it’s preinstalled on Raspberry Pi OS, but you can do the same test in a terminal, via SSH, or on a Lite version of Raspberry Pi OS if you like. Create the file with Nano and run it with:python3 <yourscript.py>
If like me, you always mix the languages syntax, download my cheat sheet for Python here!
Download now

If you get the version number on your screen, you are ready to start using OpenCV. The main goal of this tutorial was to get you there with OpenCV working in a Python script, but I’ll show you 3 examples to go a bit further.
It's a free PDF guide containing every Raspberry Pi Linux command you should know!
Download now
3 Examples to get started with OpenCV on Raspberry Pi
First script: manipulate images with OpenCV
Most projects using OpenCV are based on image manipulation. It could either be pictures stored on your computer or a live stream from a camera. OpenCV is pretty useful to load them in a script, change something and display or analyze them after that.
If you are entirely new to Python, I highly recommend reading this article first, where I explain the basics of Python on Raspberry Pi. I also think reading my book “Master Python on Raspberry Pi” is almost mandatory before trying any project with OpenCV if you don’t want to get lost quickly.
But anyway, if you have a minimal amount of experience with Python, here are a few methods you can try to get a sense of what OpenCV can do.
The first test you can do is to load a picture saved on your Raspberry Pi (it works with JPG and PNG files).
You can do this with:cv2.imread(<filename>)
Once the picture is loaded, you can use various functions included in the OpenCV library to get more data about the file, like its dimensions for example:
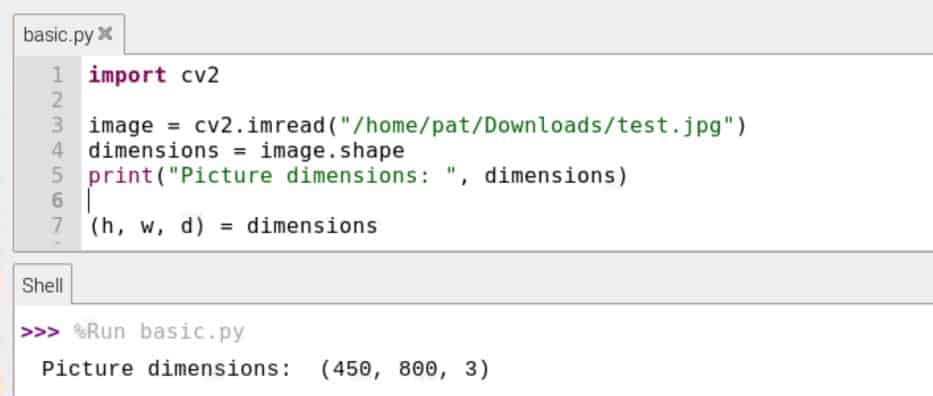
You can show the picture on the screen, by using:cv2.imshow(<window title>,<image>)
Something like:
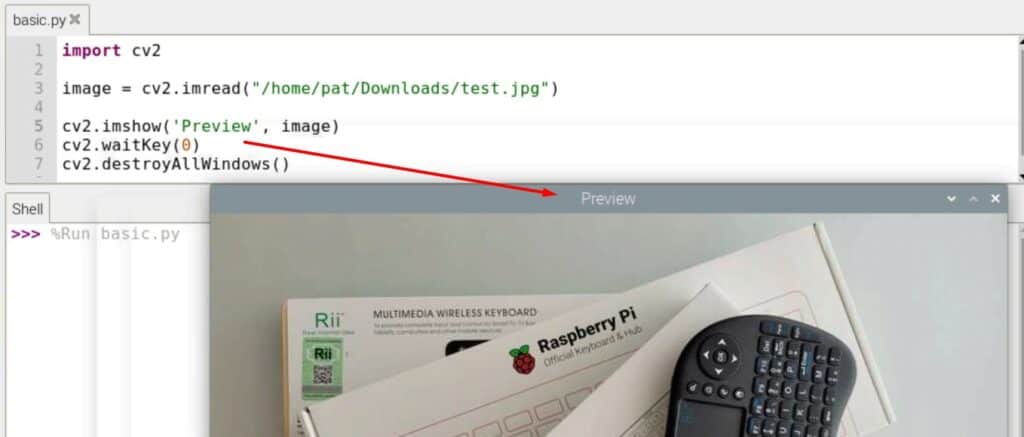
The two lines after that are used to keep the window open until you press a key. If you press “Space” for example, it will close the picture and the script will continue.cv2.waitKey(0)
cv2.destroyAllWindows()
Another useful function is the “resize” method.
You can either resize the picture to a specific size (like 200x200px in this example):cv2.resize(image, (x, y))
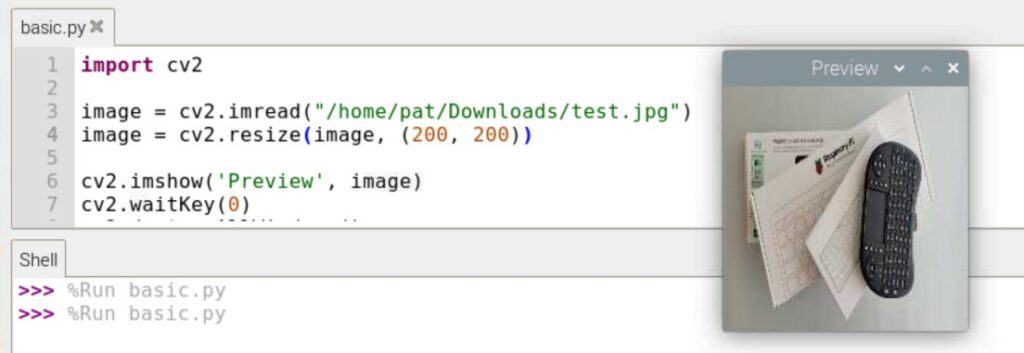
Or use a coefficient, like 50% of the original size in this example:cv2.resize(image, (0, 0), fx=X, fy=Y)
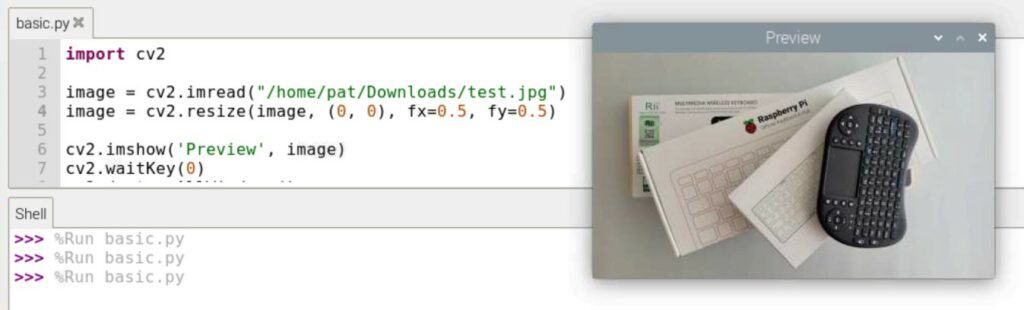
Using 0.5 means the new image will be 50% of the original size. You can use a different coefficient for width (X) and height (Y).
Finally, you can store the modified image somewhere on your Raspberry Pi, by using the “imwrite” function, like this:cv2.imwrite(<filename>, image)

Just make sure to use the same file extension and that you have access to the specified location.
These functions are a good introduction to make sure OpenCV is working properly on your system, and get used to the features you’ll use in most projects. But OpenCV can then be used with artificial intelligence (machine learning especially), the camera module, and GPIO pins to build impressive Python projects. Let’s introduce two of them.
Face recognition: use the Raspberry Pi camera
In short, the idea for that kind of project is to constantly check the camera module stream on the Raspberry Pi, and do something when a face is detected. Once trained correctly, OpenCV can not only detect a face on the camera but also recognize who it is.
Basically, you take 10 to 20 pictures of your face with the camera from various angles and then train OpenCV, so it can recognize you in a live stream. This makes many interesting projects possible, where you can code your Raspberry Pi to do something when you show up.
That was one of the examples given with the PiCar-X robot kit I tested recently. You can find the script they use to do this on the SunFounder documentation. It will draw a rectangle around the detected face, but you can do anything from there.
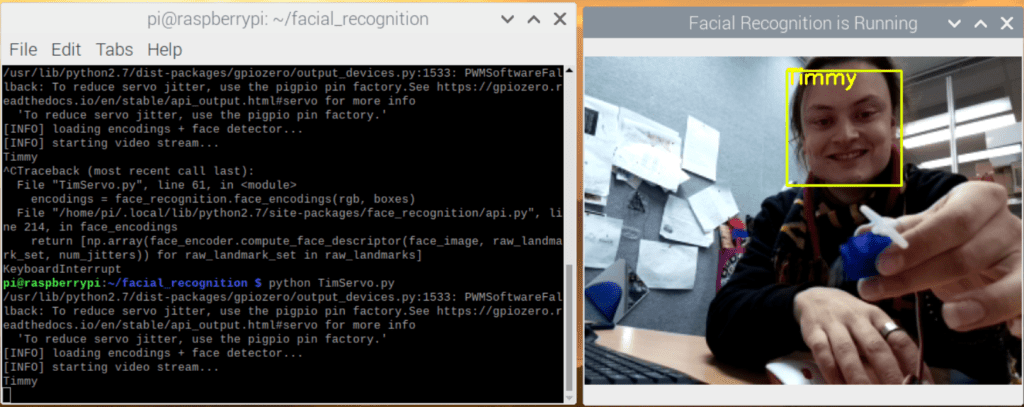
The best tutorial I have found to go a bit further on a Raspberry Pi is this one from Tim on Core Electronics. You can follow the steps or download the code for the full project directly (so you can test even if not everything is clear for you).
The idea of this simple project is to start a servo motor when his face is detected, but it can be anything (playing music? Turning the lights on in your house? There is no limit).
Warning: Most Python scripts using the camera module won’t work on the latest version of Raspberry Pi OS. It’s probably better to still use Raspberry Pi OS Buster (Legacy) to avoid these issues. Check this article for more details.
Object detection/identification
Another fun project you can try is object detection or tracking. It’s basically the same idea, but instead of detecting a face, you’ll try to identify the objects on a picture (or camera stream).
If your Raspberry Pi is mounted on a robot kit, you can even program it to follow this object (that is possible with the Robot Dog kit I also tested on this website). You have a red ball that you can throw, and the robot will follow it.
To get started, I find that this tutorial is pretty good to give you a sense of the possibilities. As with the previous project, you can either follow it step-by-step to try to understand everything or just run the full script to see it in action.
I have many other Python projects listed here if you want to experiment fun things with your Raspberry Pi. And let me know if you need more help with OpenCV, I can write step-by-step tutorials if you are interested.
It's a free PDF guide containing every Raspberry Pi Linux command you should know!
Download now
Reminder: Remember that all the members of my community get access to this website without ads, exclusive courses and much more. You can become part of this community for as little as $5 per month & get all the benefits immediately.
FAQ: OpenCV on Raspberry Pi
How to check if OpenCV is already installed on Raspberry Pi?
To check if OpenCV is installed, go to Add/Remove software and search for “OpenCV”. If some packages are installed, there will be a checkbox checked on the left. This command can also be used to list the OpenCV packages installed:sudo dpkg -l | grep "python-opencv|python3-opencv"
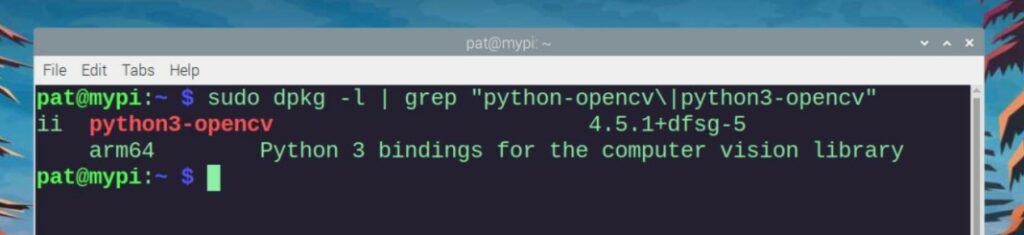
Can I use OpenCV with C++?
OpenCV developers are building it with C++, so OpenCV can be used with most languages, including C++, Java, and Python. On a Raspberry Pi (with any Debian-like distribution), OpenCV can be installed for C++ with:sudo apt install libopencv-dev
You can then start your script with:#include <opencv2/opencv.hpp>
To use OpenCV in a C++ script.
Read this article on my website if you need help to get started with C++ on Raspberry Pi.
How much memory does OpenCV use?
1 GB memory should be enough for most projects using OpenCV on a Raspberry Pi.
Obviously, the amount of memory you need will depend on what your program does (analyzing a high-quality video stream in real-time doesn’t need the same amount as resizing a small picture), but any Raspberry Pi model should be sufficient for most projects.
It may also vary depending on the distribution you use, and other applications
Whenever you’re ready, here are other ways I can help you:
The RaspberryTips Community: If you want to hang out with me and other Raspberry Pi fans, you can join the community. I share exclusive tutorials and behind-the-scenes content there. Premium members can also visit the website without ads.
Master your Raspberry Pi in 30 days: If you are looking for the best tips to become an expert on Raspberry Pi, this book is for you. Learn useful Linux skills and practice multiple projects with step-by-step guides.
The Raspberry Pi Bootcamp: Understand everything about the Raspberry Pi, stop searching for help all the time, and finally enjoy completing your projects.
Master Python on Raspberry Pi: Create, understand, and improve any Python script for your Raspberry Pi. Learn the essentials step-by-step without losing time understanding useless concepts.
You can also find all my recommendations for tools and hardware on this page.