15 Fun Raspberry Pi Projects to Experiment with Python
Python is an important brick on Raspberry Pi, and many projects can be unlocked once you know the basics of this programming language. In fact, there are so many Python projects that can be done on Raspberry Pi that it can be a bit overwhelming. That’s why I created this article, to give you a few interesting ideas to start with.
In this list you’ll discover different projects, some are for beginners, others are more advanced, some require hardware others none, and there is no specific order. Just browse and choose your next project. You can also mix several of them to create something even more interesting :).
By the way, if you get overwhelmed as soon as Python is required for a project, I recommend checking out my e-book “Master Python on Raspberry Pi“. It will guide you step-by-step to learn the essential concepts (and only the essential concepts) required to achieve any project in the future. Raspberry Pi without Python is like a car without an engine, you miss all the fun parts. Get 10% off by downloading it today!
Use the GPIO Pins
I know this may seem obvious to most of you, but I have to start with this one. Python is a popular multi-platform language. There is almost no point to use a Raspberry Pi if you don’t take advantage of the GPIO pins.
By plugging some external components to the GPIO pins, you can create much more interesting projects and take advantage of this feature on the Raspberry Pi. The basic project you’ll see in any article of this kind is to light an LED with Python. Great! That’s a mandatory project to learn to use GPIO pins with Python, but it’s no use.

GPIO pins can be used for much more than that, there are dozens of fun accessories you can plug into them! You can also use HATs if, like me, you are not the best handyman in the world.
Once you understand the basics of Python, I highly recommend starting with basic accessories. Lighting an LED yes, but also try to go a bit further and play with buttons, sensors and motors to build your own project from scratch.
Need help to get started? Check this article.
Program Minecraft Pi
I like visual things when I learn something new, and if it looks like a game, even better. Interacting with Minecraft on Raspberry Pi is perfect to learn Python.
Everything is preinstalled on Raspberry Pi OS, so you can start immediately and use the Minecraft API.

It’s maybe not the first thing you’ll do in Python, but once you know how to use functions and modules it should be a perfect exercise. It’s possible to send chat messages, interact with the blocks in-game and set blocks too (to build a house with Python for example).
I have an entire article about this, feel free to read it if you need some examples on how to get started with Python and Minecraft Pi.
Have Fun with the Sense HAT
The Sense HAT is my favorite HAT on Raspberry Pi. I have tested many HATs (list here), but the Sense HAT has many interesting features:
If like me, you always mix the languages syntax, download my cheat sheet for Python here!
Download now
- Multiple sensors: temperature, humidity, accelerometer, air pressure, etc.
- An LED matrix to display anything (texts and pixels).
- A joystick to interact with it.

It’s a product from the Raspberry Pi Foundation, so it works perfectly, and the corresponding library is pretty easy to use. I think if you can get one (check the price on Amazon), it’s a great investment. You can use it to strengthen your skills on Python but also as a building block for many other projects (for its sensors, display or both).
I have a complete tutorial on how to get started with the Sense HAT on Raspberry Pi. I recommend reading it if you are new to this. The first part explains what is the Sense Hat and how it works, and the second part is all about programming it with Python.
Create a Website with Python
I’m a web developer, and when I think of building a website, Python is not the first option that comes to me. But Python can do a good job of it, and most importantly, creating a website with Python is a great way to do something visual and concrete (learning with abstract concepts is harder and not efficient).
The easiest way to do this will be to use a framework, Flask for example. It will handle all the complicated parts of the web server, so you can just focus on the code. You’ll use Python for all the dynamic parts, but the display will be standard HTML.
They have a solid documentation here, which I recommend starting with if you are new to this. Create a few pages, link them together and share whatever you want with a few lines of Python :).
Join Our Community!
Connect, learn, and grow with other Raspberry Pi enthusiasts. Support RaspberryTips and enjoy an ad-free reading experience. Get exclusive monthly video tutorials and many other benefits.
Learn moreControl your Smart Lights with Python
This is another great way to have something visual to play with. In general, you control your smart lights from an app on your smartphone, but some of them, like Philips Hue, have a complete API. You can control them with most languages, and Python is one of them.

Whatever your goal is: creating a Christmas tree or just switching the light on and off at specific times, you can do this with Python and the Philips Hue API.
You just need a few lights (this starter kit is perfect) and the API documentation.
To make this even easier, I have a complete guide on how to do this with Philips Hue. Even if you are a beginner with Python, I think you can follow this guide and learn how to interact with the API to do exactly what you want.
Create a Graphic User Interface
Creating Python scripts is fine. But it doesn’t provide the best user experience. You have to run it manually by using a command line and if your script needs data from you to do something, you also have to use the terminal to answer the questions. This is not really a project in itself, but you can try to upgrade any of your existing projects by adding a graphic interface.
Unlike with other languages (I have bad memories of doing this in Java, maybe it’s just me), creating a graphic user interface (GUI) on Raspberry Pi can be effortless. For example, you can use the “guizero” library.
The classic “Hello world” will look like this:
from guizero import App, Text
app = App(title="Hello world")
message = Text(app, text="Hello world")
app.display()
From there, you can add input text, button, etc.
This is a great way to improve your scripts, but you can also use it to create basic games or maybe to run Linux commands in the background.
Anyway, there is a complete documentation available here, feel free to use it to get started with guizero.
If you don’t know how to install Python packages on your Raspberry Pi, click on this link to read my entire article on the topic.
Get Data from an API
API stands for “Application Programming Interface”, and it’s a method to allow two computers or programs to talk to each other. More and more websites have APIs available to get data from them, often in real-time.
So, that’s not really a Python project itself, but it’s something to think about when you have a project in mind: “Is there an API available to make this better?”.
For example, I have automated all my lights at home (I have Philips Hue lights, as explained in a previous idea). I can schedule a time to turn the lights on and off in each room. But it doesn’t work very well because you don’t need light at the same hours in January or in July. So, I connected my script to an API that provides the sunset and sunrise times, to adjust the schedule with this information. It works great!
There are many other APIs you can use, maybe you want the ISS location, the COVID stats in your country or anything else. Just do a Google Search to find what you could use.
If like me, you always mix the languages syntax, download my cheat sheet for Python here!
Download now
Build and Control your Robot with Python
Building a robot with a Raspberry Pi is like mixing several projects in one, and it’s really fun. I’m currently playing with the Robot Dog from Freenove, and it’s amazing (I have a review for this kit here). The assembly is like building a model or maybe some Lego Technic. Then you can code to control the camera, LED, ultrasonic module and obviously move the robot in all direction.
Once you understand the basics, you can set specific goals for your robot, that’s almost a new project each time. It can follow a red ball, use face recognition, and avoid obstacles.
You can read my article here if you are looking for the best robot kit for you, it’s not very expensive, and it comes with many GPIO accessories you could use for other projects.
Create a Raspberry Pi Cluster
For some demanding projects, you might think that using a Raspberry Pi is not the best choice. It may be true, the performances are not unlimited, and sometimes a small PC is a better choice. But another option could be to build a Raspberry Pi cluster.
In short, you connect several Raspberry Pi together to add their components into one more powerful entity. Then, you can use a protocol like MPI to run a Python script on all Raspberry Pi at once. There are many cases where this can be useful, as you can read in my article here.
I also have a guide on how to build a cluster of Raspberry Pi if you want to give it a try.
Create a Discord Bot with Python
Discord is a free text, voice and video messaging app. It’s similar to Slack, but was mainly oriented for gamers. It’s now used more and more in various communities, not only for gamers.
Anyway, it’s possible to use Python to build a Discord bot, which will connect to a server and perform the specific actions you program it for. In general, bots are used to add more features to the Discord server, for example: management commands, music playing, providing an API to an external device (like your Raspberry Pi).
This can be done pretty easily, as discord has a specific feature to do this (web hooks), and Python is the perfect language to use them. If you want to learn more about this, you can read this article, or watch the video below:
Note: If you want to install Discord on your Raspberry Pi, check the link to read my tutorial on the topic. There is no package directly available for ARM systems, so it’s not as straightforward as on Windows / macOS.
Build a Weather Station
This is probably not the first project you will try in Python, as you’ll need a bunch of hardware and also some decent skills in Python to control it, but It’s a popular one. With all the sensors available and the low power consumption of a Raspberry Pi, it seems like the perfect project for it.
You can find some complete kits to build this on Amazon, but you can also get all the components separately to build something custom. Coding the Python part should be complicated, as there are libraries available for each sensor. You can even power it with a solar panel as explained in this article.
The Raspberry Pi Foundation has a step-by-step project guide about this if you want (link here).
Code a Game in Python
No, coding a game is not necessarily too complicated. If you think this that’s because you have recent 3D games in mind. But a basic Tic Tac Toe game, or even an RPG-style text game can be easy to code.
Once again, the idea is to find something fun, visual and well-defined to test your skills in Python. It’s always easier to learn in this case rather than learning from abstract concepts only.
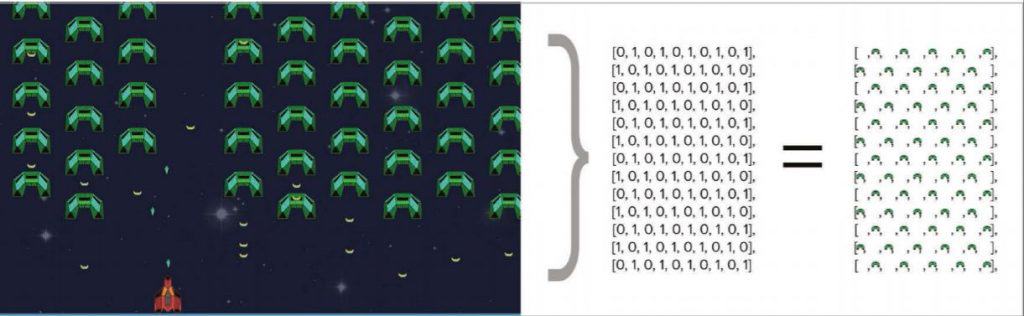
There is an entire MagPi dedicated to this, so I think it’s the best way to get started. You can read it (for free) here.
Automate your Indoor Gardening
Don’t you have green fingers? Me neither.
No worry, a Raspberry Pi and Python can save your life there!
Thanks to the GPIO pins, many projects can be done with a Raspberry Pi, and some original ones too.
You can plug a light and a pump to your Raspberry Pi, and then control it with Python to take care of your plants automatically. They will never miss sunlight and water again!
You can find one example of this kind of project on Hackster. It’s a basic one, with simple scheduling of water and light, but I’m sure we can do even better with a humidity sensor or something like that.
It's a free PDF guide containing every Raspberry Pi Linux command you should know!
Download now
Experiment with Machine Learning
If you haven’t found a project for you in this list, maybe it’s because you are already a step further!
In this case, you can dig a bit to the artificial intelligence projects. Machine learning is not always as complicated as it seems (this Cats vs Dogs project on the Raspberry Pi Foundation website is a great example).
This project uses TensorFlow to recognize cats and dogs in different images. Obviously, it can be much more complicated, but it’s a great way to learn, start small and improve your program gradually. It will be even better if you can find a real-life application, but anyway it should be fun.
You can also use OpenCV for image recognition by the way, which is another way to use your Raspberry Pi with machine learning (click on the link to read my tutorial about it).
It's a free PDF guide containing every Raspberry Pi Linux command you should know!
Download now
Want to chat with other Raspberry Pi enthusiasts? Join the community, share your current projects and ask for help directly in the forums.
Control your Camera
The Raspberry Pi has a specific slot to plug a camera module, so why not?
Yes, you can use a Python library to control your camera and do all kind of projects with it, from photo booth to timelapse or security camera.
Want more ? Check these articles:
- 11 projects you can do with your camera module on Raspberry Pi
- How to Read and Write RFID Tags With Raspberry Pi
And for more general project ideas, check out my ultimate list of suggestions here: All The Best Raspberry Pi Project Ideas (with links). You can’t go wrong with these ideas.
Whenever you’re ready, here are other ways I can help you:
The RaspberryTips Community: If you want to hang out with me and other Raspberry Pi fans, you can join the community. I share exclusive tutorials and behind-the-scenes content there. Premium members can also visit the website without ads.
Master your Raspberry Pi in 30 days: If you are looking for the best tips to become an expert on Raspberry Pi, this book is for you. Learn useful Linux skills and practice multiple projects with step-by-step guides.
The Raspberry Pi Bootcamp: Understand everything about the Raspberry Pi, stop searching for help all the time, and finally enjoy completing your projects.
Master Python on Raspberry Pi: Create, understand, and improve any Python script for your Raspberry Pi. Learn the essentials step-by-step without losing time understanding useless concepts.
You can also find all my recommendations for tools and hardware on this page.